Adding custom attribute to customers for Magento 2 extensions
To let a Magento 2 extension add a custom attribute to customers, use the following code:
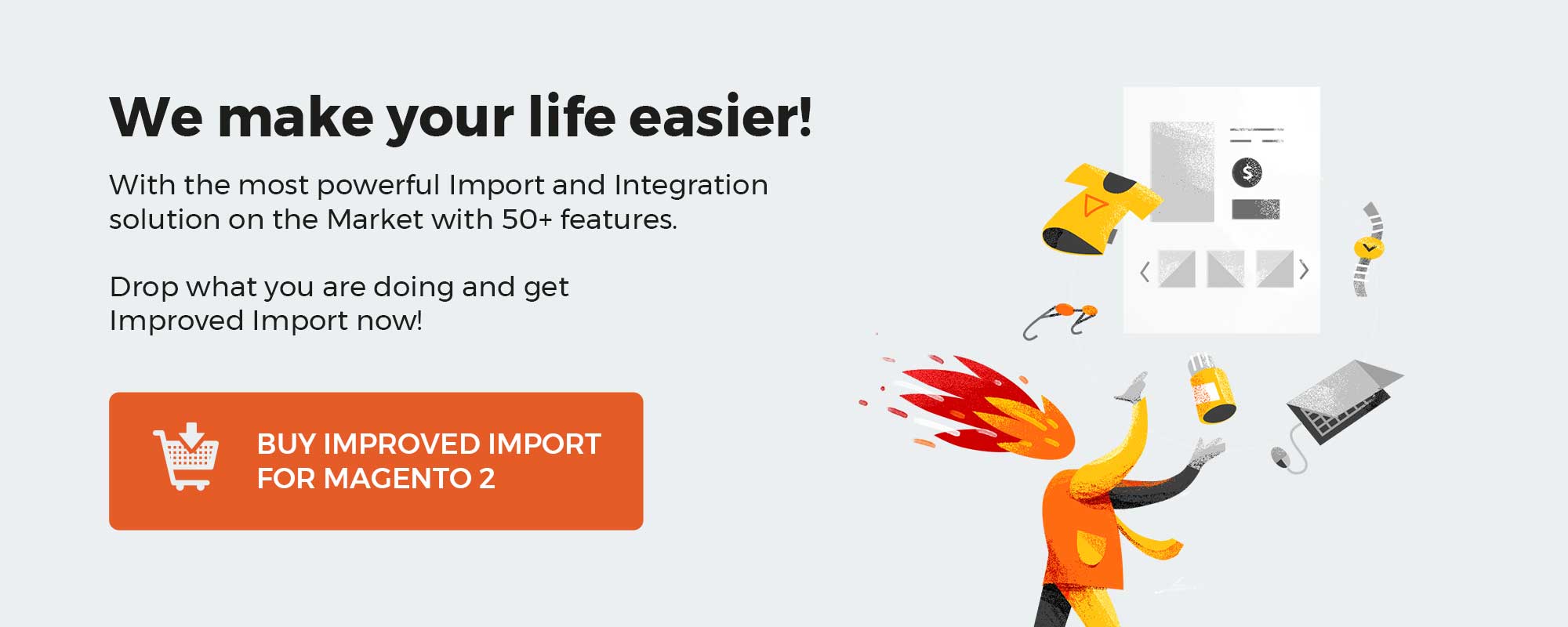
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
<?php namespace Dfe\Facebook\Setup; use Magento\Framework\Setup\InstallSchemaInterface; use Magento\Framework\Setup\ModuleContextInterface; use Magento\Framework\Setup\SchemaSetupInterface; class InstallSchema implements InstallSchemaInterface { /** * 2015-10-06 * @override * @see InstallSchemaInterface::install() * @param SchemaSetupInterface $setup * @param ModuleContextInterface $context * @return void */ public function install(SchemaSetupInterface $setup, ModuleContextInterface $context) { $setup->startSetup(); $setup->getConnection()->addColumn(rm_table('customer_entity'), self::F__FACEBOOK_ID, 'varchar(25) DEFAULT NULL' ); $setup->endSetup(); } /** * @used-by \Dfe\Facebook\Setup\InstallSchema::install() * @used-by \Dfe\Facebook\Setup\InstallData::install() */ const F__FACEBOOK_ID = 'dfe_facebook_id'; } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
<?php namespace Dfe\Facebook\Setup; use Magento\Framework\Setup\InstallDataInterface; use Magento\Framework\Setup\ModuleContextInterface; use Magento\Framework\Setup\ModuleDataSetupInterface; class InstallData implements InstallDataInterface { /** * 2015-10-06 * @override * @see InstallDataInterface::install() * @param ModuleDataSetupInterface $setup * @param ModuleContextInterface $context * @return void */ public function install(ModuleDataSetupInterface $setup, ModuleContextInterface $context) { df_eav()->addAttribute('customer', InstallSchema::F__FACEBOOK_ID, array( 'type' => 'static', 'label' => 'Facebook ID', 'input' => 'text', 'sort_order' => 1000, 'position' => 1000, 'visible' => false, 'system' => false, 'required' => false )); /** @var int $attributeId */ $attributeId = rm_first(rm_fetch_col( 'eav_attribute', 'attribute_id', 'attribute_code', InstallSchema::F__FACEBOOK_ID )); rm_conn()->insert(rm_table('customer_form_attribute'), array( 'form_code' => 'adminhtml_customer', 'attribute_id' => $attributeId )); } } |
1 2 3 4 |
/** * @return \Magento\Eav\Setup\EavSetup' */ function df_eav() {return df_o('Magento\Eav\Setup\EavSetup');} |
It is necessary to pay extra attention to the visible parameter that becomes the value of the is_visible column from the customer_eav_attribute table.
Set it to true and the attribute will be displayed in the admin customer form.
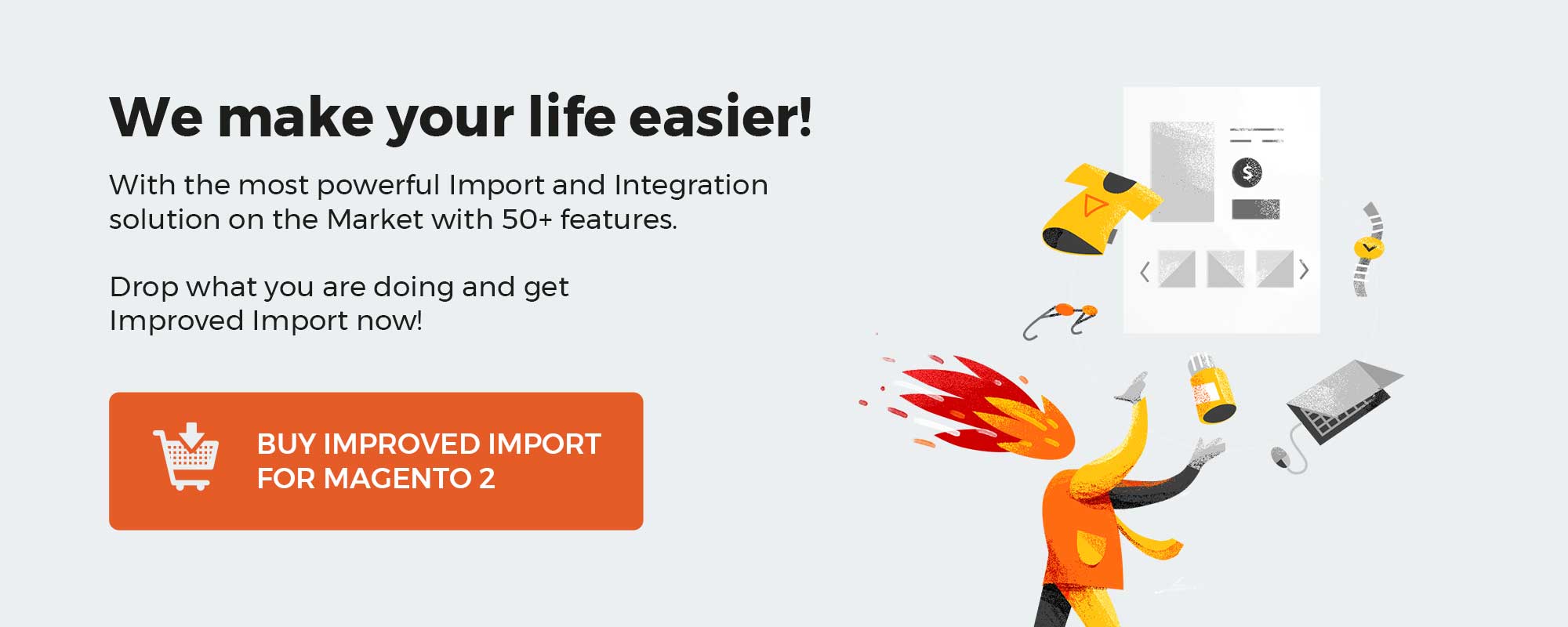