How to return a JSON response from a controller in Magento 2
Below, we describe how to return a JSON response from a controller in Magento 2.
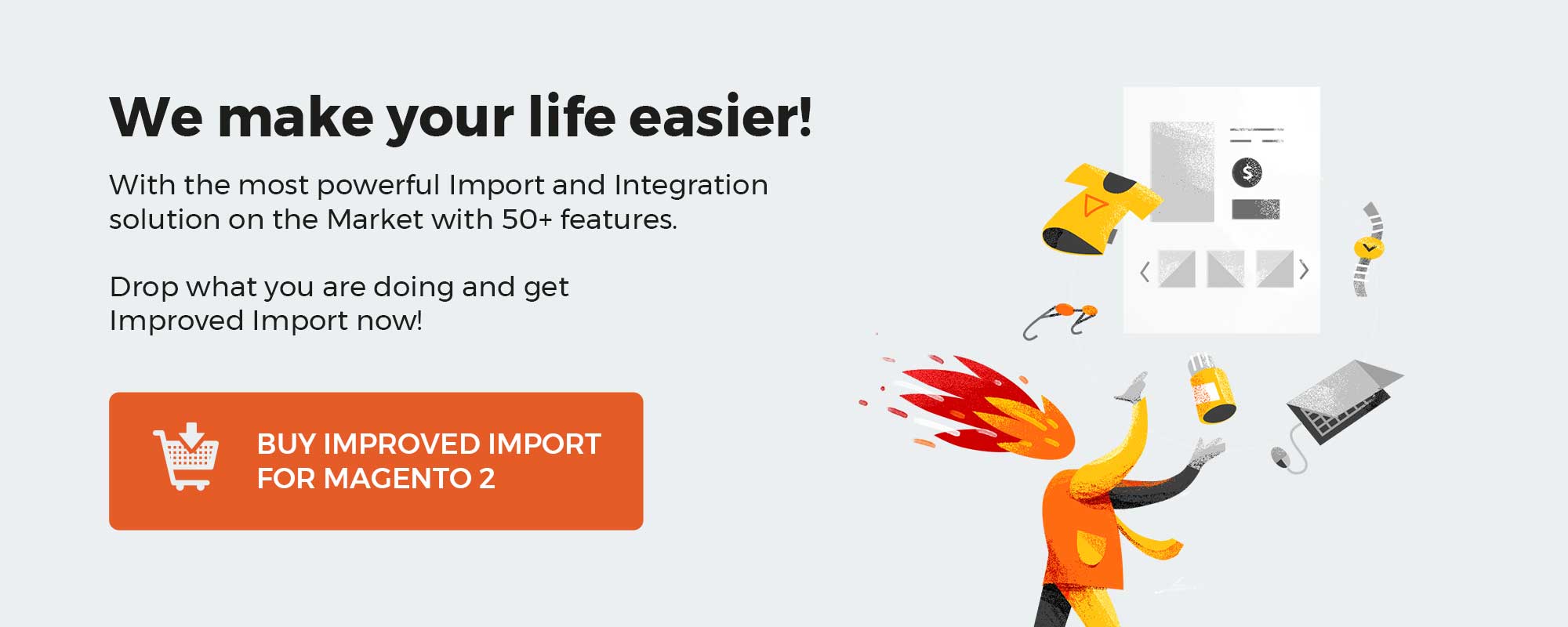
Dmitry Fedyuk provides the following example of Magento 2 backend controller with JSON response:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
<?php namespace Dfe\Login\Controller\Adminhtml\DfeLogin; class Google extends \Magento\Backend\App\AbstractAction { /** * @param \Magento\Backend\App\Action\Context $context * @param \Magento\Framework\Controller\Result\JsonFactory $resultJsonFactory */ public function __construct( \Magento\Backend\App\Action\Context $context ,\Magento\Framework\Controller\Result\JsonFactory $resultJsonFactory ) { parent::__construct($context); $this->resultJsonFactory = $resultJsonFactory; } /** * @return \Magento\Framework\Controller\Result\Json */ public function execute() { /** @var \Magento\Framework\Controller\Result\Json $result */ $result = $this->resultJsonFactory->create(); return $result->setData(['success' => true]); } } |
etc/adminhtml/routes.xml:
1 2 3 4 5 6 7 8 9 |
<?xml version='1.0'?> <config xmlns:xsi='http://www.w3.org/2001/XMLSchema-instance' xsi:noNamespaceSchemaLocation='../../../../../../lib/internal/Magento/Framework/App/etc/routes.xsd'> <router id='admin'> <route id='adminhtml' frontName='admin'> <module name='Dfe_Login' before='Magento_Backend' /> </route> </router> </config> |
And these are examples from the Magento 2 core:
Table of contents
- 1 magento/magento2/blob/8fd3e8/app/code/Magento/Backend/Controller/Adminhtml/Ajax/Translate.php#L38-L58
- 2 magento/magento2/blob/8fd3e8/app/code/Magento/Catalog/Controller/Adminhtml/Category/CategoriesJson.php#L36-L41
- 3 agento/magento2/blob/8fd3e8/app/code/Magento/Catalog/Controller/Adminhtml/Category/CategoriesJson.php#L48-L64
- 4 magento/magento2/blob/8fd3e8/app/code/Magento/Customer/Controller/Ajax/Login.php#L135-L143
- 5 magento/magento2/blob/8fd3e8/app/code/Magento/Customer/Controller/Ajax/Login.php#L159-L162
- 6 magento/magento2/blob/8fd3e8/app/code/Magento/Customer/Controller/Ajax/Login.php#L191-L193
magento/magento2/blob/8fd3e8/app/code/Magento/Backend/Controller/Adminhtml/Ajax/Translate.php#L38-L58
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
/** * Ajax action for inline translation * * @return \Magento\Framework\Controller\Result\Json */ public function execute() { $translate = (array)$this->getRequest()->getPost('translate'); /** @var \Magento\Framework\Controller\Result\Json $resultJson */ $resultJson = $this->resultJsonFactory->create(); try { $this->inlineParser->processAjaxPost($translate); $response = ['success' => 'true']; } catch (\Exception $e) { $response = ['error' => 'true', 'message' => $e->getMessage()]; } $this->_actionFlag->set('', self::FLAG_NO_POST_DISPATCH, true); return $resultJson->setData($response); } |
magento/magento2/blob/8fd3e8/app/code/Magento/Catalog/Controller/Adminhtml/Category/CategoriesJson.php#L36-L41
1 2 3 4 5 6 |
/** * Get tree node (Ajax version) * * @return \Magento\Framework\Controller\ResultInterface */ public function execute() |
agento/magento2/blob/8fd3e8/app/code/Magento/Catalog/Controller/Adminhtml/Category/CategoriesJson.php#L48-L64
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
$categoryId = (int)$this->getRequest()->getPost('id'); if ($categoryId) { $this->getRequest()->setParam('id', $categoryId); $category = $this->_initCategory(); if (!$category) { /** @var \Magento\Backend\Model\View\Result\Redirect $resultRedirect */ $resultRedirect = $this->resultRedirectFactory->create(); return $resultRedirect->setPath('catalog/*/', ['_current' => true, 'id' => null]); } /** @var \Magento\Framework\Controller\Result\Json $resultJson */ $resultJson = $this->resultJsonFactory->create(); return $resultJson->setJsonData( $this->layoutFactory->create()->createBlock('Magento\Catalog\Block\Adminhtml\Category\Tree') ->getTreeJson($category) ); } |
magento/magento2/blob/8fd3e8/app/code/Magento/Customer/Controller/Ajax/Login.php#L135-L143
1 2 3 4 5 6 7 8 9 |
/** * Login registered users and initiate a session. * * Expects a POST. ex for JSON {"username":"user@magento.com", "password":"userpassword"} * * @return \Magento\Framework\Controller\ResultInterface * @SuppressWarnings(PHPMD.CyclomaticComplexity) */ public function execute() |
magento/magento2/blob/8fd3e8/app/code/Magento/Customer/Controller/Ajax/Login.php#L159-L162
1 2 3 4 |
$response = [ 'errors' => false, 'message' => __('Login successful.') ]; |
magento/magento2/blob/8fd3e8/app/code/Magento/Customer/Controller/Ajax/Login.php#L191-L193
1 2 3 |
/** @var \Magento\Framework\Controller\Result\Json $resultJson */ $resultJson = $this->resultJsonFactory->create(); return $resultJson->setData($response); |
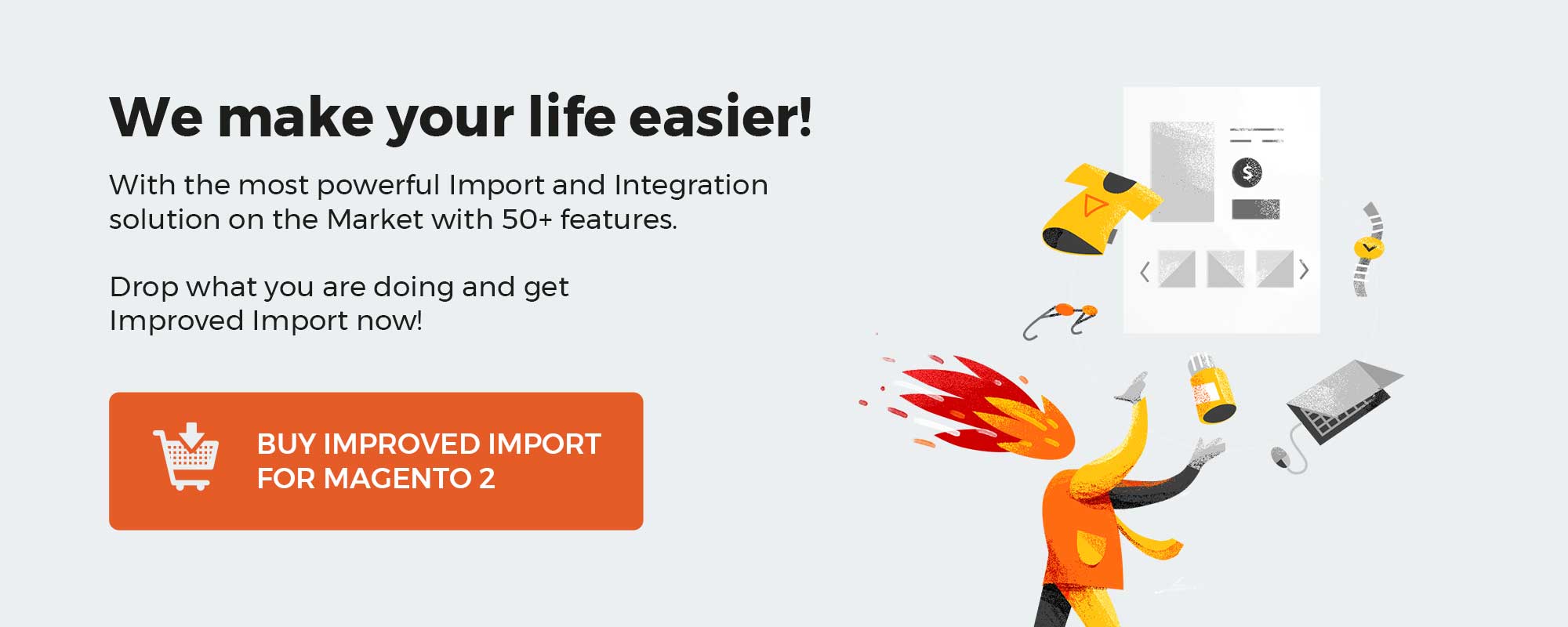