HTTP Error 429 Explained: Why ‘Too Many Requests’ Happens and How to Fix It
When you encounter the HTTP Error 429, it means the server is telling you: “Slow down, you’re sending too many requests.” The 429 error “Too Many Requests” is a rate-limiting response that indicates you have exceeded the allowed number of requests in a given period. This issue often arises when using APIs or while web scraping, but it can also affect everyday users visiting your e-commerce website. In this guide, we’ll dive deep into what causes the 429 error code, explore common scenarios where it appears, and, most importantly, explain how to fix error 429 Too Many Requests effectively. You will also find a chapter dedicated to the HTTP Error 429 in e-commerce.
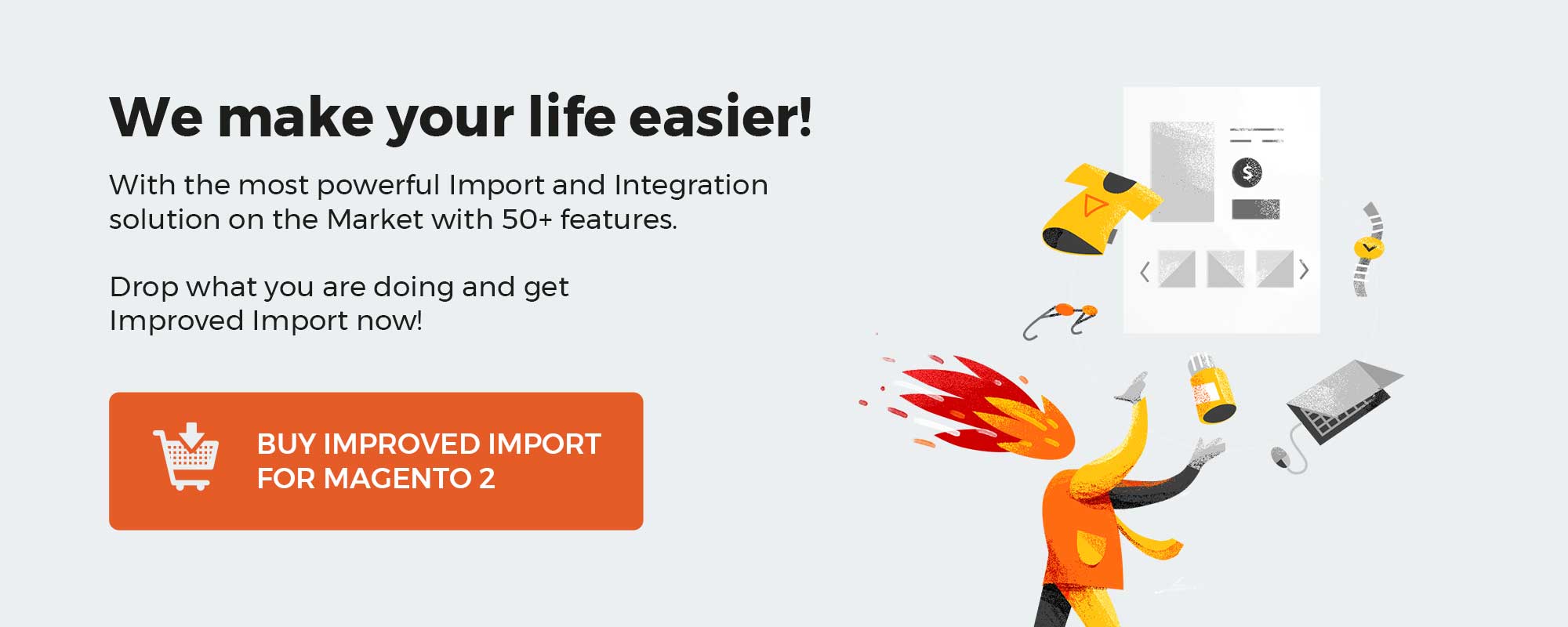
Table of contents
What Is HTTP Error 429: Too Many Requests?
The HTTP 429 status code indicates that a client has sent too many requests in a given amount of time. It’s a rate-limiting response that servers use as a protective measure. Let’s explore why it occurs and what threats it helps servers defend against.
Why Does the HTTP 429 Error Happen?
The 429 error occurs when a user or application exceeds the rate limit imposed by the server. This rate limit may be based on:
- Number of requests per second (e.g., more than 100 requests per second).
- Daily request limits (e.g., a maximum of 10,000 requests per day).
- Burst limits (e.g., exceeding a limit during a sudden spike in traffic).
- Concurrent requests (e.g., sending too many simultaneous requests).
This error is most common with APIs and services that need to manage heavy traffic and protect their infrastructure from overload.
Why Do Servers Use HTTP 429 Rate Limiting?
Here are the main reasons why servers trigger the HTTP 429 Too Many Requests error:
- Prevent Server Overload: High traffic, especially from automated scripts or bots, can overwhelm servers, making them slow or unresponsive. By returning a 429 error, the server protects itself from overload, ensuring it can handle requests from legitimate users.
- API Rate Limiting: Many services, like Twitter, Google, and GitHub, enforce request limits on their APIs to ensure fair usage. For example, GitHub’s API may allow up to 60 requests per hour for unauthenticated users. Once this limit is hit, the server responds with an HTTP 429 error, asking the client to wait before making more requests.
- Mitigating DDoS Attacks: Distributed Denial of Service (DDoS) attacks flood a server with requests to exhaust its resources. Implementing a rate limit and responding with a 429 status code helps servers identify and block malicious traffic early.
- Preventing Brute-Force Attempts: Many websites use the 429 error to limit login attempts. If a user submits several incorrect login attempts in quick succession, the server may respond with a 429 status code to block further attempts temporarily, protecting against brute-force attacks.
What Does the HTTP Error 429 Response Look Like?
A typical 429 response from a server looks like this:
1 2 3 4 5 6 7 8 9 |
HTTP/1.1 429 Too Many Requests Content-Type: application/json Retry-After: 60 { "error": "Too Many Requests", "message": "You have exceeded the rate limit. Please wait before making more requests.", "code": 429 } |
Its key components include:
- Status Code (429): Indicates that the request cannot be processed because of rate limits.
- Retry-After Header: Suggests when the client can try making requests again. This value can be in seconds (e.g., Retry-After: 120 meaning wait 2 minutes) or as a specific date/time (e.g., Retry-After: Thu, 16 Nov 2024 10:00:00 GMT).
- Error Message: Provides additional information to help users understand why the error occurred. (e.g., “You have exceeded the rate limit. Please wait before making more requests.”)
Common Use Cases for Error Code 429
Below, we explore common use cases for error 429:
API Rate Limiting
If you’re building an application that interacts with third-party APIs, you might encounter a 429 error if your app makes too many requests in a short period. Most APIs have clear rate limits defined in their documentation. For example:
- GitHub’s API may limit unauthenticated users to 60 requests per hour.
- Twitter’s API may limit certain endpoints to 15 requests per 15 minutes.
If the user exceeds these limits, they will receive an HTTP 429 error with a Retry-After header indicating when they can try again.
1 2 3 4 5 6 7 |
GET https://api.example.com/data HTTP/1.1 429 Too Many Requests Retry-After: 120 { "message": "Rate limit exceeded. Please try again in 2 minutes." } |
Web Scraping
When scraping websites, sending too many requests too quickly may trigger HTTP 429 errors as the site protects itself against automated abuse.
If a scraper is configured to make 10 requests per second, but the website allows only 5 requests per second, the scraper may receive a 429 error after exceeding the limit.
Introduce delays or use exponential backoff to reduce the request rate.
Login Rate Limiting
To prevent brute-force attacks, websites may limit the number of login attempts in a short time.
Suppose a user tries to log in 5 times within a minute. If the site allows only 3 login attempts per minute, the next attempt will return a 429 error with a Retry-After header.
1 2 3 4 5 6 |
HTTP/1.1 429 Too Many Requests Retry-After: 30 { "error": "Too many login attempts. Please wait 30 seconds and try again." } |
How to Fix Error 429 Too Many Requests
Now, let’s explore four easy steps to handle the HTTP Error 429:
1. Follow the Retry-After Header: When the server responds with a Retry-After header, respect the waiting time before making another request.
1 2 3 4 5 6 7 8 9 |
import time import requests response = requests.get("https://api.example.com/data") if response.status_code == 429: retry_after = int(response.headers.get("Retry-After", 60)) print(f"Rate limit exceeded. Retrying after {retry_after} seconds.") time.sleep(retry_after) response = requests.get("https://api.example.com/data") |
2. Implement Exponential Backoff: Instead of retrying immediately after a 429 error, use exponential backoff, which increases the wait time after each failed attempt, reducing the risk of further errors.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
import time retry_attempt = 0 max_attempts = 5 while retry_attempt < max_attempts: response = requests.get("https://api.example.com/data") if response.status_code == 429: wait_time = 2 ** retry_attempt print(f"Rate limit exceeded. Waiting for {wait_time} seconds.") time.sleep(wait_time) retry_attempt += 1 else: break |
3. Reduce the Request Frequency: If you encounter 429 errors frequently, consider decreasing the frequency of your requests to align with the server’s rate limits.
4. Optimize API Usage: Use caching or batch requests to reduce the number of API calls. For example, instead of requesting data individually for each item, try fetching data in bulk.
How HTTP Error 429 Impacts E-Commerce Platforms: Magento, Shopify, and Shopware
In the world of e-commerce, the HTTP error 429 can disrupt both the customer experience and backend operations. When platforms like Magento, Shopify, and Shopware hit this rate limit error, it can affect a range of functionalities, from product searches to inventory updates. Let’s explore how the HTTP 429 error appears on these platforms and why it matters for online retailers.
Why HTTP 429 Error Happens in E-Commerce
E-commerce platforms heavily rely on APIs for various tasks such as:
- Synchronizing Inventory: APIs connect to third-party tools like inventory management systems to keep stock levels updated.
- Handling High Traffic: During sales events, customers may generate a high volume of simultaneous requests (e.g., product searches, checkout processes).
- Integrating with Third-Party Services: Payment gateways, shipping providers, and analytics tools often require API calls.
If these requests exceed the allowed rate limit, the server responds with a 429 error, temporarily blocking further requests.
Error 429 on Magento
Magento, especially when extended with third-party plugins, can generate a large number of API requests. Here’s how the 429 error can impact Magento stores:
- Product Synchronization Delays: If your Magento store syncs data with ERP or CRM systems too frequently, it may exceed the API rate limits, causing product data updates to fail.
Solution: Implement request throttling or use caching to reduce the frequency of API calls. - High-Traffic Events: Magento stores often experience traffic spikes during promotions or sales, which can overwhelm the server and trigger the 429 error.
Solution: Use a Content Delivery Network (CDN) and load balancers to handle increased traffic, reducing the likelihood of triggering rate limits. - Third-Party Extension Conflicts: Some Magento extensions make frequent API calls to external services (e.g., for currency rates or product recommendations). If not optimized, they can quickly reach the API limits.
Solution: Configure rate limits and adjust API call frequency in the extension settings, or consider replacing overly aggressive plugins.
Error 429 on Shopify
Shopify enforces strict API rate limits to maintain performance across its shared infrastructure, especially since many Shopify stores use apps that rely on API calls.
- Rate Limits for Apps: Shopify has a 2 requests per second limit for the Admin API. Exceeding this rate can result in a 429 error, causing apps to fail in syncing data or processing orders.
Solution: Developers should implement throttling and retry logic based on the Retry-After header returned by Shopify’s API. - Checkout and Payment Issues: During high-demand periods, like flash sales, customers making simultaneous purchases may trigger API rate limits, leading to a poor checkout experience.
Solution: Use Shopify’s built-in features like Shopify Plus API enhancements, which offer higher rate limits for large-scale stores. - Data Import and Export: Importing large product catalogs or updating multiple orders via API can easily hit Shopify’s rate limits, resulting in delays.
Solution: Break data import into smaller batches and monitor the API response for Retry-After headers to avoid 429 errors.
Error 429 on Shopware
Shopware, a flexible platform often used by European e-commerce businesses, may also encounter 429 errors, particularly with API-heavy configurations.
- API-Driven Integrations: Shopware stores often use APIs to connect with payment providers, analytics tools, and external marketplaces (e.g., Amazon or eBay). These integrations can generate high request volumes, especially during synchronization tasks.
Solution: Use Shopware’s API rate limit configuration options to fine-tune the request frequency and implement error-handling mechanisms. - Performance During Sales Peaks: Similar to Magento and Shopify, Shopware stores can experience high traffic during promotions, leading to rate limit issues.
Solution: Optimize your Shopware store by enabling caching and load balancing and distributing API calls across multiple nodes to avoid overwhelming a single server. - Frequent Data Sync Issues: Extensions and plugins in Shopware may not always handle API limits effectively, causing frequent 429 errors during tasks like bulk product updates.
Solution: Choose extensions with built-in rate-limiting features or implement a custom retry mechanism based on Shopware’s API response headers.
Best Practices for Handling HTTP 429 Errors on E-Commerce Platforms
Follow these best practices to handle the error 429 on e-commerce platforms:
- Monitor API Usage: Regularly check your API usage and analyze logs for patterns that trigger 429 errors. Tools like Google Analytics or built-in monitoring features in Shopify and Magento can help you identify issues.
- Implement Exponential Backoff: Instead of immediately retrying a failed request, increase the wait time after each attempt. This reduces the chance of hitting the rate limit repeatedly.
- Use Caching and Throttling: Reduce the frequency of API calls by caching responses and implementing throttling in your code, especially for non-critical data.
- Communicate with Third-Party Service Providers: If your store relies heavily on third-party APIs, discuss your needs with the service provider. Some providers offer higher rate limits for premium accounts or can suggest optimizations.
- Plan for High-Traffic Events: Prepare for traffic spikes during sales or promotions by optimizing your store’s performance and testing your API limits beforehand.
Final Thoughts
The HTTP error 429 “Too Many Requests” is a protection mechanism for servers against overuse. It signals clients to slow down their request rate, ensuring fair access and preventing abuse. Proper handling of this error can improve the stability of both the client application and the server, especially when interacting with high-traffic APIs or websites.
For e-commerce platforms like Magento, Shopify, and Shopware, the error 429 is a signal that your store is making too many requests in a short period. Proper handling of this error is essential to maintain a smooth shopping experience and reliable backend operations. By optimizing API usage, implementing retry logic, and preparing for peak traffic, you can avoid disruptions caused by this common rate-limiting error.
FAQ
What is HTTP 429?
The HTTP error 429, known as “Too Many Requests,” is a status code that indicates the client has sent too many requests in a short period. This response is used by servers to enforce rate limits and prevent overloading. It often includes a “Retry-After” header, which tells you how long to wait before sending more requests.
How do I fix error 429?
To fix the HTTP error 429, first check the “Retry-After” header and wait the specified time before retrying. Reduce your request frequency, use caching, and implement exponential backoff in your code to gradually increase wait times. If the issue persists, consider contacting the service provider to request a higher rate limit.
What is the difference between HTTP 429 and 503?
The error 429 means the client has sent too many requests and exceeded the server’s rate limit. It is typically accompanied by a “Retry-After” header. The HTTP 503 error, on the other hand, indicates that the server is temporarily unable to handle the request due to maintenance or overload, and may not include a “Retry-After” header.
How to avoid HTTP 429 errors?
To avoid HTTP 429 errors, limit the number of requests you send per second, respect the rate limits outlined by the API, use caching to avoid redundant requests, and implement exponential backoff in your request strategy. Additionally, batching API calls can help reduce the total number of requests.
How many HTTP requests are too many?
The number of HTTP requests considered “too many” depends on the server’s rate-limiting policy. APIs often allow between 60 to 1000 requests per hour, while some services limit traffic to 10-20 requests per second. Always refer to the specific API documentation for the exact limits to avoid triggering the error 429.
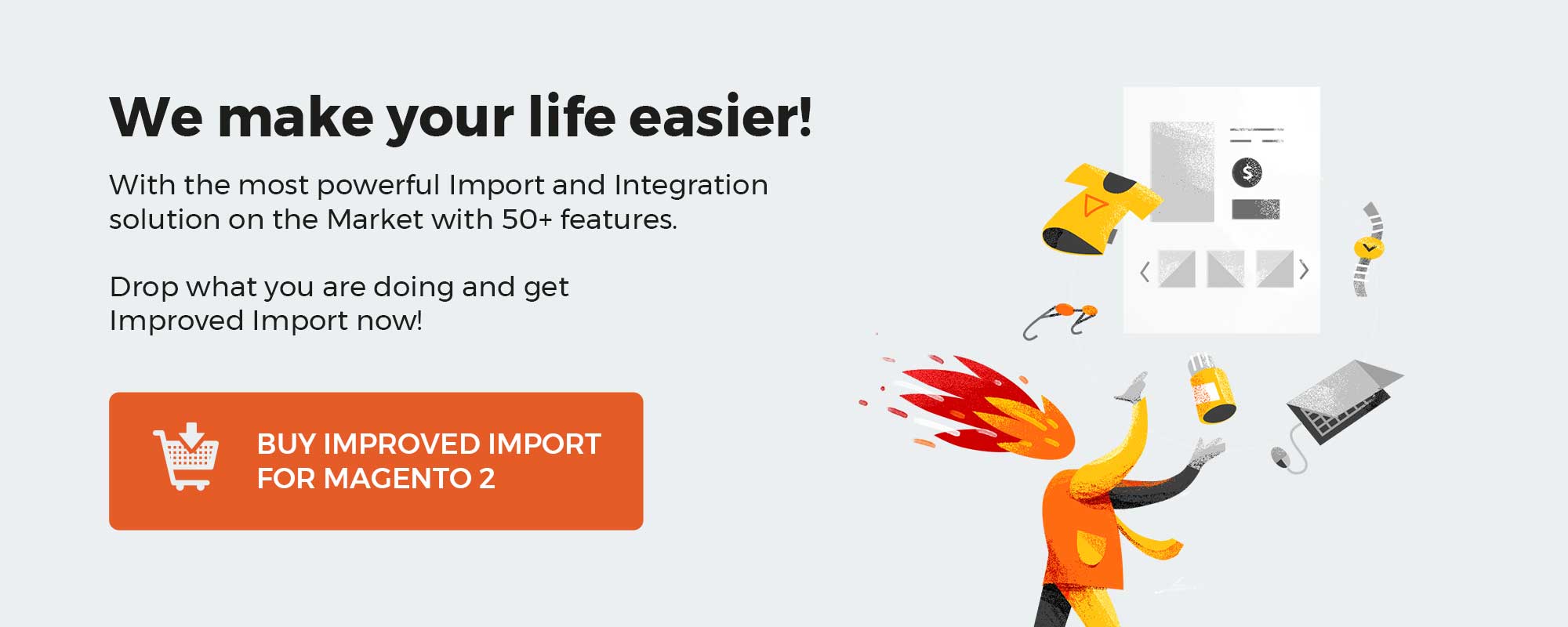