How to create custom helper in Magento 2
First of all, you should create the app/code/Company1/Module1/composer.json file in order to create custom helper in Magento 2:
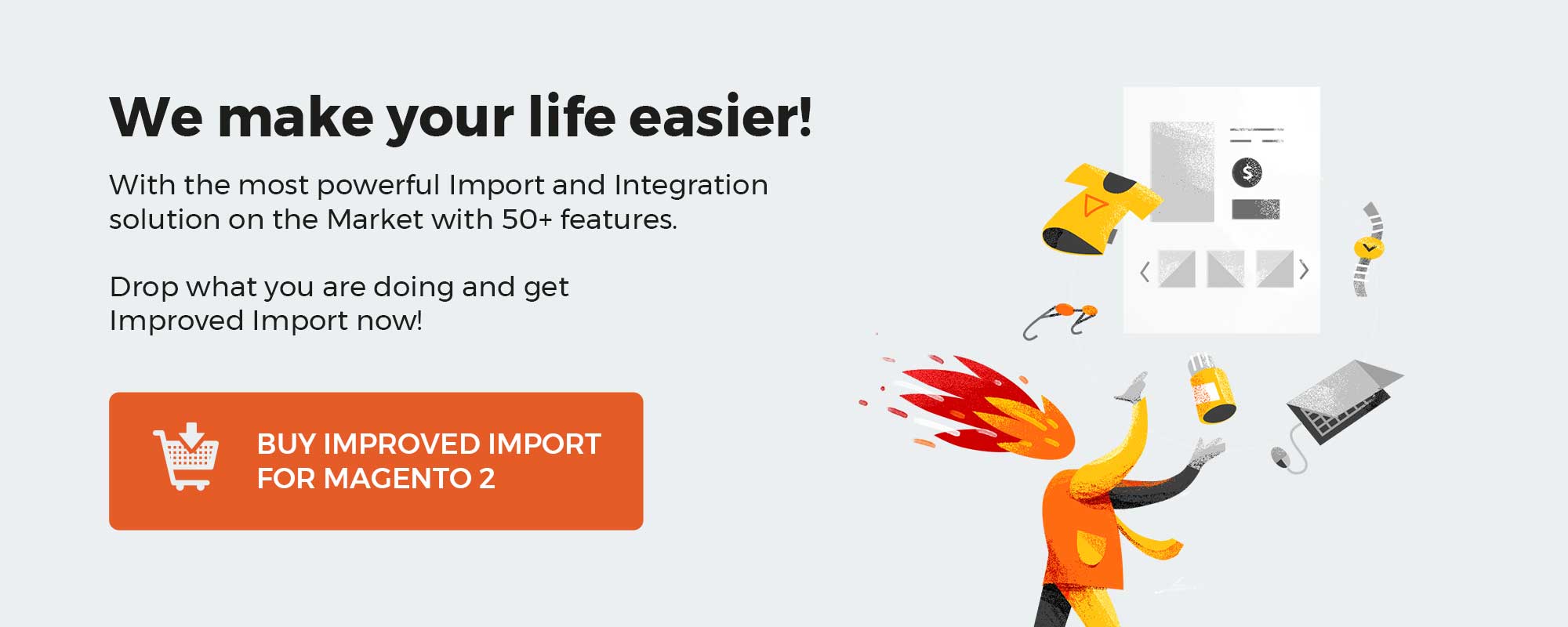
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
{ "name": "company1/module-module1", "description": "", "require": { "php": "~5.5.0|~5.6.0|~7.0.0", "magento/framework": "100.0.*", "magento/module-ui": "100.0.*", "magento/module-config": "100.0.*", "magento/module-directory": "100.0.*" }, "type": "magento2-module", "version": "100.0.0", "license": [ "OSL-3.0", "AFL-3.0" ], "autoload": { "files": [ "registration.php" ], "psr-4": { "Company1\\Module1\\": "" } } } |
Then, create the app/code/Company1/Module1/registration.php file
1 2 3 4 5 |
\Magento\Framework\Component\ComponentRegistrar::register( \Magento\Framework\Component\ComponentRegistrar::MODULE, 'Company1_Module1', __DIR__ ); |
And make app/code/Company1/Module1/etc/module.xml
1 2 3 4 5 6 7 8 |
<?xml version="1.0"?> <config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd"> <module name="Company1_Module1" setup_version="2.0.0"> <sequence> <module name="Magento_Directory"/> </sequence> </module> </config> |
You’ve just created a module, and finally it is necessary to create a helper class inside the Helper folder. Create app/code/Company1/Module1/Helper/Data.php
1 2 3 4 5 6 7 8 9 |
namespace Company1\Module1\Helper; class Data extends \Magento\Framework\App\Helper\AbstractHelper { public function someMethod() { return 1; } } |
Use it inside controller as follows:
1 |
$this->_objectManager->create('Company1\Module1\Helper\Data')->someMethod(); |
Inside the block:
1 2 3 4 5 6 7 8 |
public function __construct( \Magento\Framework\View\Element\Template\Context $context, \Company1\Module1\Helper\Data $helper array $data = [] ) { $this->helper = $helper parent::__construct($context, $data); } |
As you can see, $this->helper is an instance of Data. To overwrite classes, use preference.
app/code/Company1/Module1/etc/di.xml
1 2 3 4 |
<xml version="1.0"?> <config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:ObjectManager/etc/config.xsd"> <preference for="Magento\Directory\Helper\Data" type="Company1\Module1\Helper\Data" /> </config> |
Alternatively, you can also use plugin, since it is the best way to avoid rewrite conflict. For further information, examine
Source (
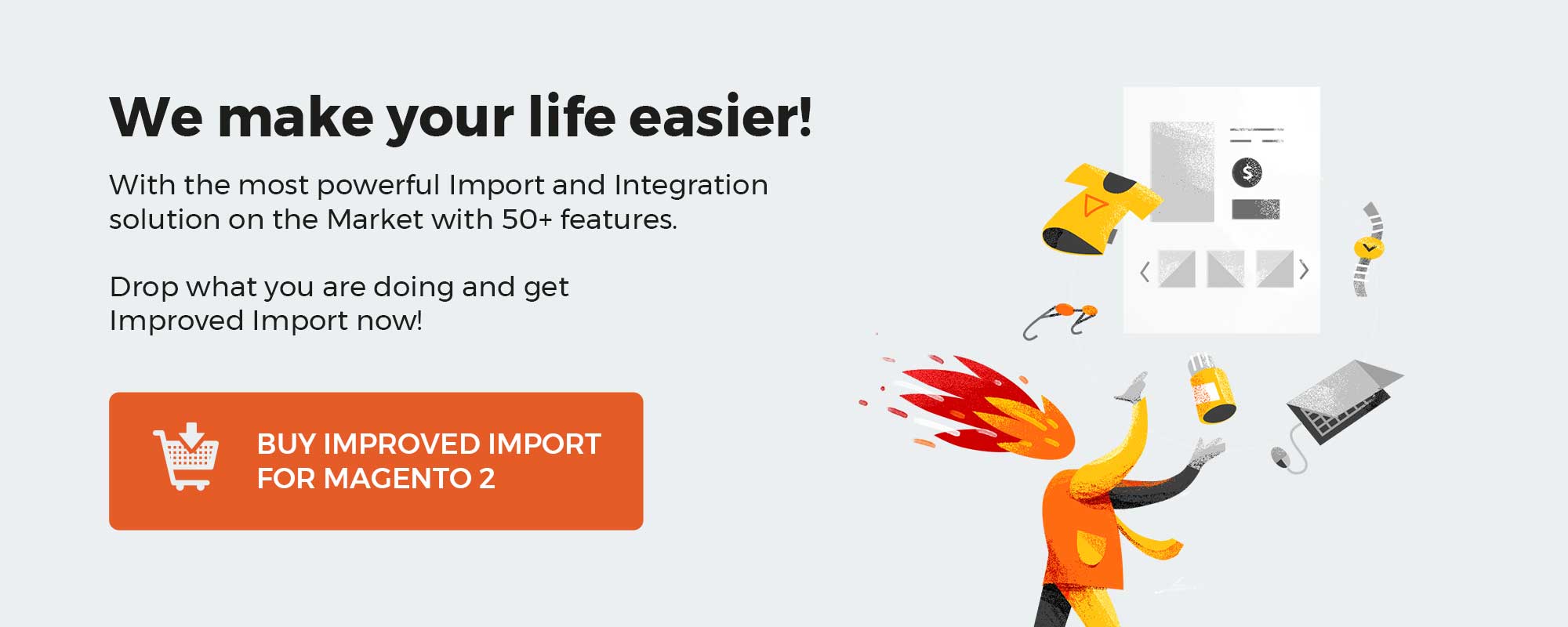